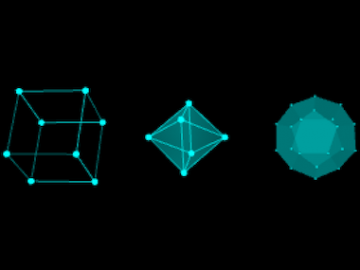
I couldn’t find a complete implementation of the five Platonic Solids in Processing, so I decided to put some Processing classes together. You can find them here:
The Five Platonic Solids in Processing
As shown in the image above, the classes have the added possibility of drawing polyhedra vertices with custom radius (an example sketch is included in the GitHub project).
The classes were initially inspired by the Icosahedron for Processing by Ira Greenberg, with a little help from the Processing Forum, specifically the lists of vertices (unit vectors) for each of the solids:
Tetrahedron: PVector[] tetrahedron = { new PVector( 1, 1, 1 ), new PVector(-1, -1, 1 ), new PVector(-1, 1,-1 ), new PVector( 1, -1, -1 ) }; Octahedron: PVector[] octahedron = { new PVector( 1, 0, 0 ), new PVector( 0, 1, 0 ), new PVector( 0, 0, 1 ), new PVector( -1, 0, 0 ), new PVector( 0, -1, 0 ), new PVector( 0, 0, -1 ) }; Hexahedron: PVector[] hexahedron = { new PVector( 1, 1, 1 ), new PVector( -1, 1, 1 ), new PVector( -1, -1, 1 ), new PVector( 1, -1, 1 ), new PVector( 1, 1, -1 ), new PVector( -1, 1, -1 ), new PVector( -1, -1, -1 ), new PVector( 1, -1, -1 ) }; Icosahedron: float _ = 0.525731; float __ = 0.850650; PVector[] icosahedron = { new PVector(-_, 0, __), new PVector(_, 0, __), new PVector(-_, 0, -__), new PVector(_, 0, -__), new PVector(0, __, _), new PVector(0, __, -_), new PVector(0, -__, _), new PVector(0, -__, -_), new PVector(__, _, 0), new PVector(-__, _, 0), new PVector(__, -_, 0), new PVector(-__, -_, 0) }; Dodecahedron: float _ = 1.618033; //golden mean float __ = 0.618033; PVector[] dodecahedron = { new PVector(0, __, _), new PVector(0, __, -_), new PVector(0, -__, _), new PVector(0, -__, -_), new PVector(_, 0, __), new PVector(_, 0, -__), new PVector(-_, 0, __), new PVector(-_, 0, -__), new PVector(__, _, 0), new PVector(__, -_, 0), new PVector(-__, _, 0), new PVector(-__, -_, 0), new PVector(1, 1, 1), new PVector(1, 1, -1), new PVector(1, -1, 1), new PVector(1, -1, -1), new PVector(-1, 1, 1), new PVector(-1, 1, -1), new PVector(-1, -1, 1), new PVector(-1, -1, -1) };